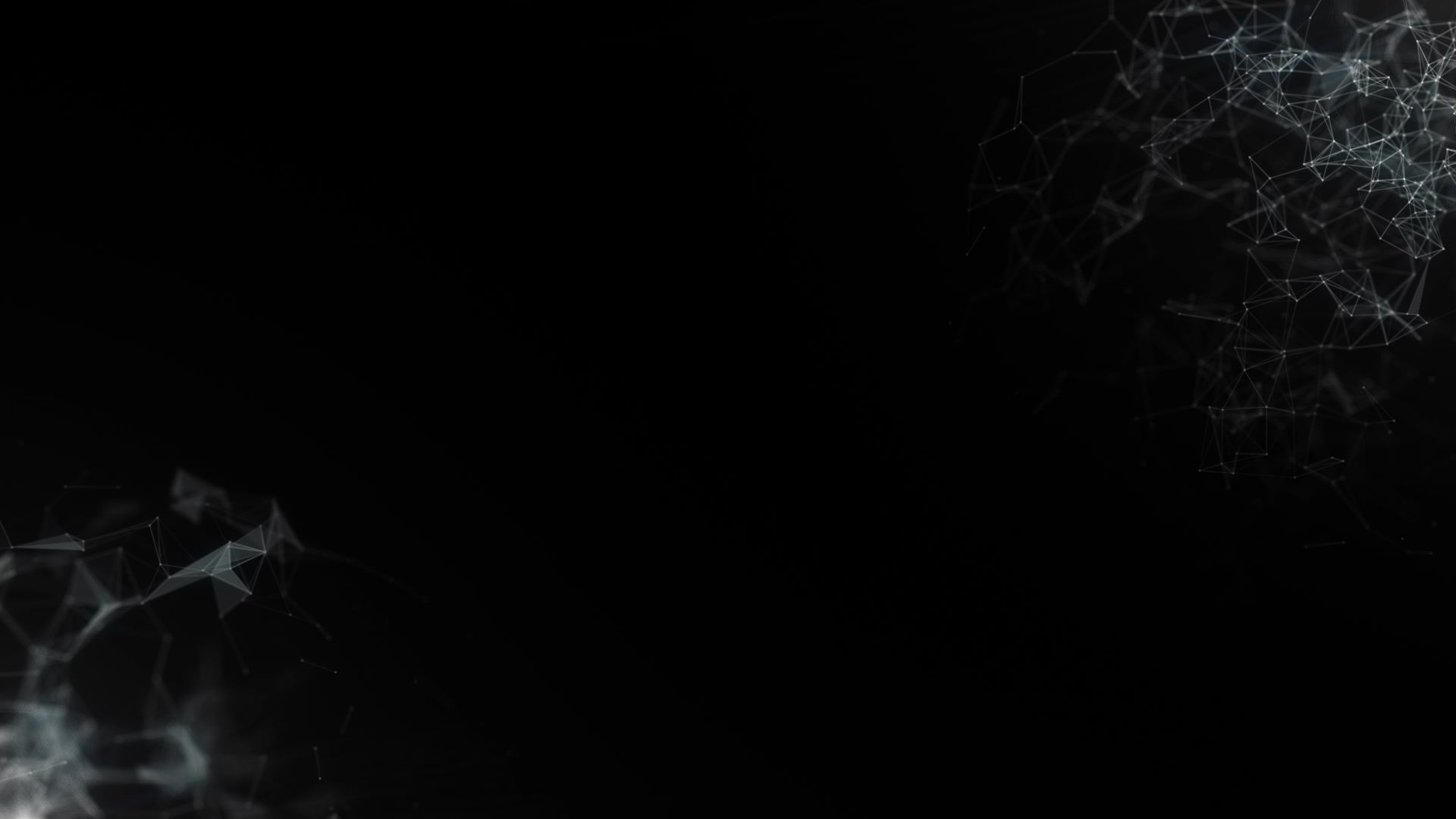
Object Oriented Programming
Classes are a 'blueprint'. They allow you to repeatedly call on an object as well as its attributes. It is a way of programming that saves time and prevents you from repeating code too much. Classes are made up of objects, attributes and methods. The screenshots in this blog are from w3schools.com.
​
​
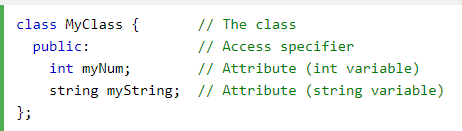
In this example, the class command is used to create a class. Inside this, a string and integer variable are declared. When variables are declared within a class they are called attributes.
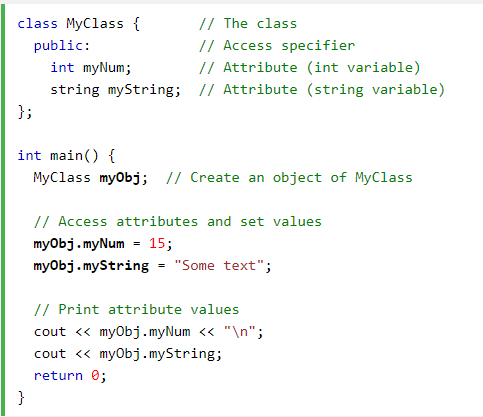
Here, the object myObj is created. The integer and string attributes are then assigned to the object and printed.
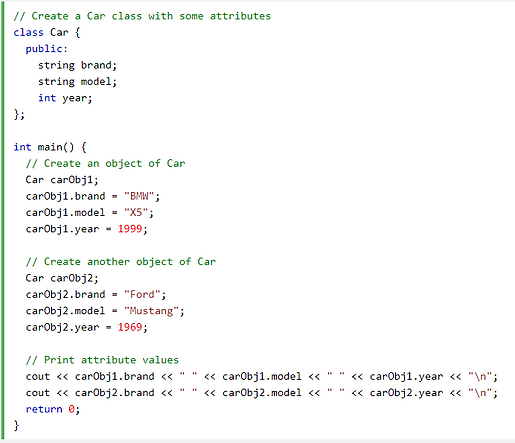
This demonstrates how one class can be used for multiple objects using the same attributes with different assignments.
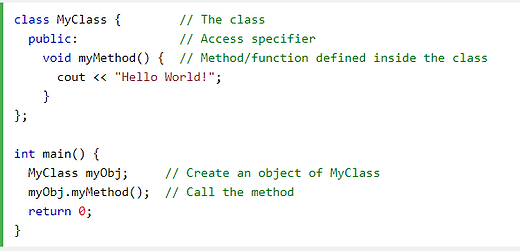
This example uses methods. Methods can be defined inside or outside of their class. This example defines it inside of the class named myMethod.

Defining a method outside of a class requires you to first declare it inside the class and define it outside. So, at the top myMethod is created inside of MyClass. It is then defined using MyClass::myMethod() to print 'hello world!'.
Access Specification
Attributes and methods can be public or private. Using the public: or private: keywords specifies which one it is. Public access allows the attribute/method to be used outside the class and private restricts it to only inside the class and it cannot be called on outside of it.
Encapsulation
Private variables can be used to keep certain data away from users. Private elements can be edited by users using public get and set methods.
Inheritance
Attributes and methods can be 'inherited' from one class to another. The class that inherits another is called the derived class and the class being inherited from is called the base class.

In this example, the car class inherits the attributes and methods from the vehicle class. Using inheritance allows you to reuse attributes and methods of an existing class when you make a new one.
​
Inheritance can be repeated, so a class that has inherited from another can also be inherited from. This is called multilevel inheritance.
​
Inheritance also has its own access specifier called protected. It is the same as private, but it can also be accessed in inherited classes.
Inheritance
Polymorphism is a way to use inherited methods from another class. This allows you to do one action in multiple different ways.

The class Animal has a method called animalSound.(). The class Pig : public Animal line creates a derived class that inherits the method from Animal and also uses the animalSound method, but with a different output.